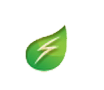
SOURCE CODE

// Libraries
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
#include "DHT.h"
int value;
String pumpstate = "";
// Credentials
String deviceId = "xxxxxxxxxxxxxxxxx"; // * set your device id (will be the MQTT client username)
String deviceSecret = "xxxxxxxxxxxxxxxxxxxxxxxxxx"; // * set your device secret (will be the MQTT client password)
String clientId = "soil moisture sensor"; // * set a random string (max 23 chars, will be the MQTT client id)
// WiFi name & password
const char* ssid = "HOM"; //NAME WIFI
const char* password = "Gravitech2014"; //PASSWORD WIFI
// Topics
String outTopic = "devices/" + deviceId + "/set"; // * MQTT channel where physical updates are published
String inTopic = "devices/" + deviceId + "/get"; // * MQTT channel where lelylan updates are received
// WiFi client
WiFiClient wifiClient;
// Pin & type
#define PIN_VCC 16
#define PIN_GND 13
#define DHTPIN 14
#define DHTTYPE DHT22
// Initialize DHT sensor
DHT *dht;
// MQTT server address
IPAddress server(178, 62, 108, 47);
// MQTT
PubSubClient client(server);
void setup() {
Serial.begin(115200);
delay(500);
pinMode(4,OUTPUT);
pinMode(PIN_VCC, OUTPUT);
pinMode(PIN_GND, OUTPUT);
digitalWrite( PIN_VCC, HIGH );
digitalWrite( PIN_GND, LOW );
dht = new DHT( DHTPIN, DHTTYPE, 15 );
dht->begin();
// Connect to WiFi
Serial.println();
Serial.println();
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
lelylanConnection(); // MQTT server connection
}
void loop() {
// Keep connection open
lelylanConnection();
// Reading temperature and humidity
// int h = dht->readHumidity();
// Read temperature as Celsius
int t = dht->readTemperature();
value = analogRead(A0); //Read analog signal
Serial.println(value); //Print value to Serial Port
delay(1000); //delay for 1 second
if (value > 800) // ถ้าค่าอุณหภูมิมากกว่า 800 ให้สั่งการไปยัง RELAY เปิด
{ digitalWrite(4, LOW);
pumpstate = "open";
}
else { digitalWrite(4, HIGH); //ถ้าค่าอุณหภูมิน้อยกว่า 800 ให้สั่งการไปยัง RELAY ปิด
pumpstate = "close";
}
// Messages for MQTT
String humidity = "{\"properties\":[{\"id\":\"559ccfc7b4f3840925000009\",\"value\":\"" + String(t) + "\"}]}";
String temperature = "{\"properties\":[{\"id\":\"55a0ea7f68969a79af000008\",\"value\":\"" + String(value) + "\"}]}";
String pump = "{\"properties\":[{\"id\":\"55a0ea9368969a81a6000004\",\"value\":\"" + pumpstate + "\"}]}"; //ส่งค่าสถานะ RELAY แปลงเป็น STRING ขึ้นเว็บ LELYLAN
// Publish temperature
client.publish(outTopic, (char *) temperature.c_str());
delay(1000);
// Publish humidity
client.publish(outTopic, (char *) humidity.c_str());
delay(1000);
client.publish(outTopic, (char *) pump.c_str());
delay(1000);
}
/* MQTT server connection */
void lelylanConnection() {
// add reconnection logics
if (!client.connected()) {
// connection to MQTT server
if (client.connect(MQTT::Connect(clientId)
.set_auth(deviceId, deviceSecret))) {
Serial.println("[PHYSICAL] Successfully connected with MQTT");
lelylanSubscribe(); // topic subscription
}
}
client.loop();
}
/* MQTT subscribe */
void lelylanSubscribe() {
client.subscribe(inTopic);
}
/* Receive Lelylan message and confirm the physical change */
void callback(char* topic, byte* payload, unsigned int length) {
}
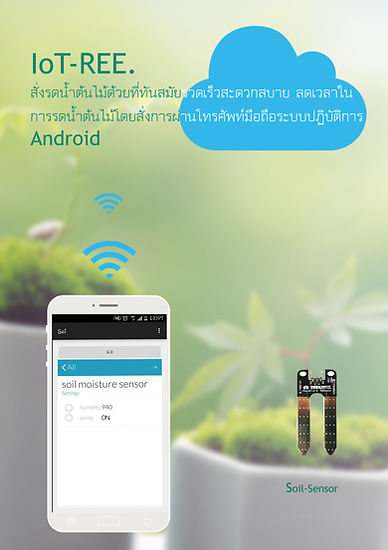